In this tutorial we will learn how to use the uROS library for micropython: https://github.com/mgtm98/uRos
ROS is an Operating System for Robots in which the different “nodes” communicate with a central server through TCP messages in JSON format.
This library allows us to implement a ROS node in our SBC. It will connect to a server and will be able to send (publish) or receive (subscribe to) messages.
The code to post a message is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | import network from uros import * from uros.std_msgs import std_Bool lan = network.LAN() lan.active(True) lan.ifconfig( ( "10.0.0.1", "255.255.255.0", "10.0.0.2", "8.8.8.8" ) ) while( lan.status() != 3 ): print( ".", end="" ) time.sleep(1) print( "Connected!" ) uRos = Ros( "10.0.0.2", 9090 ) #creating new uRos object msg = std_Bool( data = True ) #creating a Ros string msg object uRos.publish( "uRosTopic", msg ) #publishing the msg object print("done") |
In this code we first connect to the LAN network and then to the ROS server; we define the format of our message and finally we publish.
On the PC we execute a code in python that acts as a ROS server. For simplicity, this code is just a socket server that prints the data to the console.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 | import socket import sys def socket_server( host, port ): s = socket.socket(socket.AF_INET, socket.SOCK_STREAM) s.bind((host, port)) s.listen(1) while True: conn, addr = s.accept() print('Connected by', addr) while True: data = conn.recv(1024) if not data: break print(data) conn.close() print("conn closed") s.close() print("s closed") socket_server( '10.0.0.2', 9090 ) print("done") |
As a result, the data received on the server is displayed:
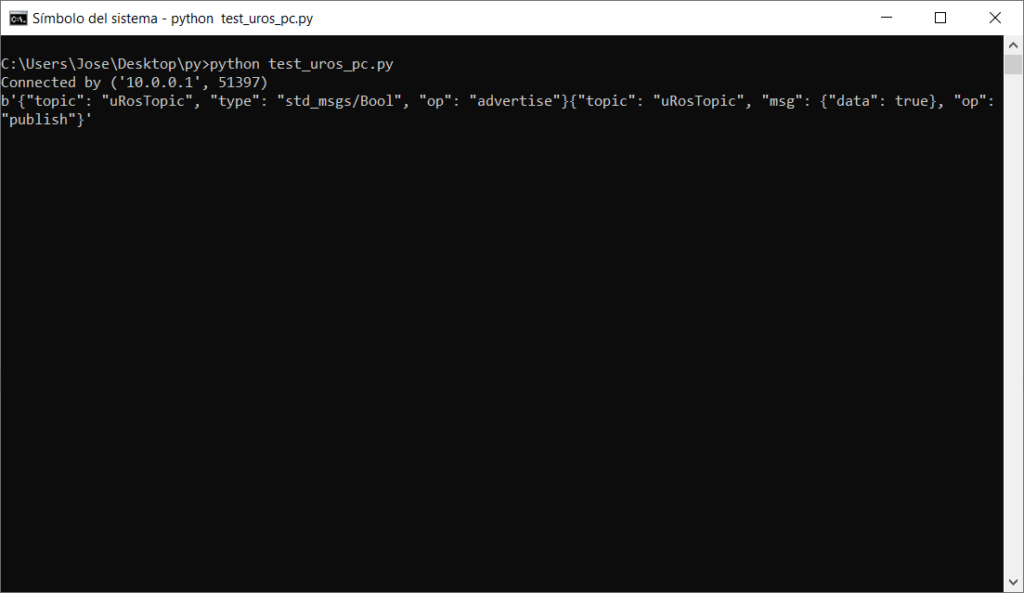
If we examine the data we see two parts:
1. The definition of our uRosTopic message. This is only sent once for each new type of message.

2. And the published data. This is sent every time we publish a new value.

Now you are ready to connect your SBC to any ROS device via ethernet, define a custom message and send it with only few lines of python code.